React Best Practices Every Developer Have to Follow in 2024
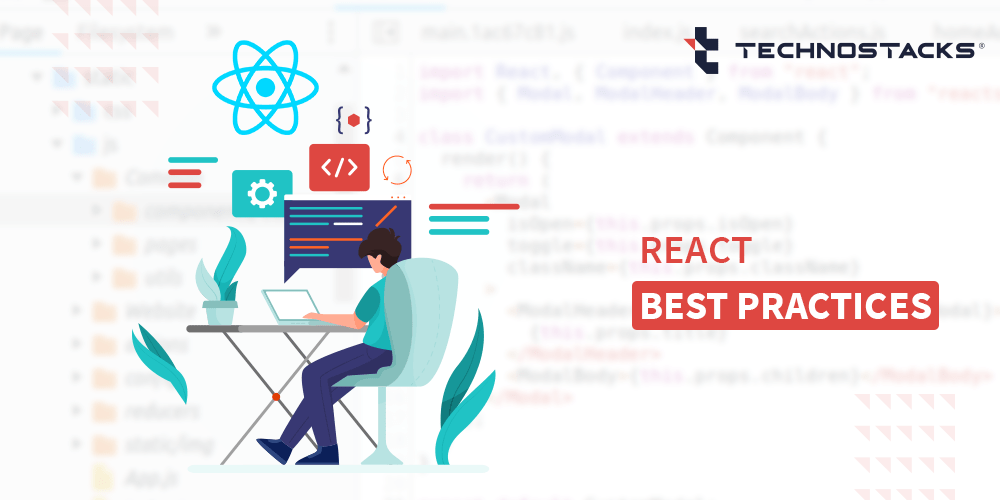

Technostacks
ReactJS serves as a universally accepted and well-known platform when it comes to frontend frameworks. However, React Js is useful to 60 percent of the developers, so there is a need to learn about the best strategies.
ReactJS is a supple open-source JavaScript library utilized to build exceptional applications. In this article, you will highlight the insights and react best practices to help React Js developers and businesses create great and high-performing applications.
List of React Js Best Practices to Follow in the Year 2024
- Create a new base react application structure
To follow the best practices for React projects, it is necessary to create an ascending structure of the project. For example, the NPM command-based create-react-app can be used, and the React structure varies depending on the project’s complexity and requirements.While creating a react project, there is a need to define a scalable project structure which is important for the best react practices for a robust project. It is possible to use the NPM command ‘create-react-app.’ React folder structure differs based on project specification and complexity. You will get the availability of the various React Js best practices considered while defining project architecture:First of all, there is a need for the Folder Layout.The React folder structure architecture focuses on reusable components to share the design pattern among multiple internal projects. The idea of component-centric file structure implies that all the components’ files (like test, CSS, JavaScript, assets, etc.) must fall under a single folder.Components | --Login | --tests-- --Login.test.js --Login.jsx --Login.scss --LoginAPI.js
Also, there is another approach to grouping the file types.
APIs | --LoginAPI --ProfileAPI --UserAPI Components | --Login.jsx --Login.test.js --Profile.jsx --Profile.test.js --User.jsx
Also, there is a possibility for nesting them based on requirements.
- CSS in JS
When it comes to large projects, one of the most fundamental of the React best practices is styling and theming. However, it turns out to be a challenging task like maintaining those big CSS files. So, this is where the CSS-in-JS solutions came into the picture.Similar to managing those enormous CSS files, designing and theming can be a complex effort in a larger project. Thus, the idea of CSS-in-JS solutions (i.e., embedding CSS in JavaScript) emerged. Diverse libraries are based on this idea.Among numerous libraries, you can use the needed one based on the requirement, like some for the complicated themes. - Children Props
Across precise JSX expressions with an opening and a closing tag, the content amid these tags gets conceded as a distinct prop: props.children. It serves as a part of the React documentation and props.children works as the special prop, automatically passed to every component. The goal is to render the content included between the opening and closing tags when invoking a component.Also, it finds use in the rendering method of the content of one component inside another component. That said, there is a possibility to pass functions as child props.Stack Overflow’s 2020 Developer Survey suggests that from 57.378 replies, 68.9% of responses showed interest in enduring engagement with React JS.One of the most valuable components of React is the capability to receive and render child properties. It makes it easy and effortless to create reusable components. All you need to do is enclose props. children with some markup or action:function house(props) { return <h3> Hello { props.children }!</h3>; } function room() { return ( <> <h1>How Are You?</h1> <house type="duplex" /> </> ); }
- Reusability principle in React
Reusable components of the react developer serve as the piece of UI used in various parts of an application that can help build more than the UI instance. Also, you can get the button component displayed with different colors in several parts of the application. Therefore, reusability is important, and this is why there is a need to create new components to the minimum required.Rule of one function = one component, improve the reusability of components. Skip is trying to build a new component for a function when you see that there is a component for that function. Reusing components across your project not only achieves consistency but also contributes to the community.The UI utilized in various portions of an application help in building more than the UI instance is a reusable component of the react developer.Read More:- Best React Component LibrariesIn case you notice that any component becomes huge and difficult to maintain, break it up into as many smaller components. For example, create a Button component that can handle icons. You should ensure that making it more modular to cover many cases with the same piece of code. Make sure that the components should be abstract enough but not overly complex. Here is an example:class IconButton extends React.Component { [...] render() { return ( <button onClick={this.props.onClick()}> <i class={this.props.iconClass}></i> </button> ); } }
- Consolidation of duplicate code
The Don’t Repeat Yourself principle is the best guide for preventing duplication. Look for similarities and patterns in the code to accomplish the goal.Consolidation of duplicated code serves as one of the react js best practices. A common rule for all code is keeping it brief and concise. The best way to avoid duplication is following Don’t Repeat Yourself (DRY). Achieve the objective by scrutinizing the code for patterns and similarities. With that, you will get the scope to eliminate duplication. Rewriting can make it more concise.Also, this condition is largely dependent on the reusability principle in React. Also, if you wish to add multiple buttons that contain icons, as an alternative to adding the markup for each button, use the Icon Button component. Also, go further by mapping everything into an array.const buttons = ['facebook', 'twitter', 'youtube']; return ( <div> { buttons.map( (button) => { return ( <IconButton onClick={doStuff( button )} iconClass={button} /> ); } ) } </div> );
- Higher-Order Components (HOC)
React’s advanced methodology enables the reuse of component functionality within the render technique. A component can be changed to a high order by using an advanced level of the component.Consideration of higher-order components as one of the best practices for Reactjs developers. Higher-Order Components serve as an advanced technique in React, allowing reuse of the component logic inside the render method. When considering the advanced level, transform a component into a higher order of the component. For example, show some components when the user stays logged in and complement the similar code with every component.Read More:- Reasons Why Use React.js For Web Development - Stop relying on class-based components
The ideal practice is to stop using class-based components in your React application. Instead, your components can be written in React as class-based components.The best practice to follow for your React application is to Stop relying on class-based components. React will allow writing your components as class-based components. This is the major reason why the Java/C# developers lean toward writing classes.However, class-based components have an issue in the form that they start becoming complex and harder for you and your teammates to understand. In addition to that, these components are less abstract. The introduction of React hooks has become a boon for the developers as they are no longer required to write classes. Including useState, useEffect, and use context can assist you in the objective. - Embracing the useState hook
It is always a good idea to develop a custom hook whenever you need to reuse stateful logic previously implemented in another functional component.Usually, there is a need for the class-based components to maintain the state. However, this condition is no longer true with useState.React components are immutable in general as there are associations with the performance metrics. In this regard, it can be said that the useState hook provides the best scope to re-render a component after changing a variable.import { useState } from ‘react’; export function Foo() { const [ someData, setSomeData ] = useState(“”); return ( <> <p>{ someData }</p> <button onClick={() => setSomeData(Math.random())}>Go</button> </> }
The above code shows that setSomeData serves as a function that will re-draw this component after assigning the someData variable. Thus, when the user clicks on <button>, there will be a need for putting in some random number in someData and then redraw.
- Naming the component after the function
To distinguish components from other JSX files that aren’t components, you must always name them in PascalCase. TextField, NavMenu, and SuccessButton are a few examples.Naming the component is considered as the best react practices and it should bear the potential to convey instantly what the component does. Naming the component based on the need for the code confuses you at a future point. Name a component Avatar that finds use anywhere.It will be highly favorable for authors, users, or in comments. So, let us spot the difference. AuthorAvatar can also be used in the context of its usage. But, in this case, the issue will be that it can limit the utility of that component. Naming a component after the function will turn out to be useful to the community. - Using capitals for component names
Capitalized — component names with the first letter uppercase are handled as React components (example, Foo />); Dot Notation – component names with an explicit dot in the name, irrespective of the specific case, are treated as React components as well.Using JSX (a JavaScript extension) means that the names of the components need to begin with uppercase letters. Let us take an example here. You can choose to name components as SelectButton instead of the select button. This is essential as it gives an easy path to the JSX to identify them differently from default HTML tags.Earlier React versions used to have a list of all built-in names to differentiate them from custom names. The limitation, in that case, was, however, that it needed constant updating. If you see that JSX is not your language, you can use lowercase letters. But the problem still stays the same. It comes with loads of difficulty in the reusability of components.Read More:- React vs Angular - Minding the other naming conventions
The term “naming a component” refers to the name we assign to the class or const that defines the component:class MyComponent extends Component { } const MyComponent () => {};
When working with React, the developers are generally using JSX (JavaScript Extension) files. The general rule states that any component you create for React must be used in the Pascal case or upper camel case. For example, in case you wish to create a function that submits a form, name it SubmitForm in the upper camel case. The goal here is to not use submitForm, submit_form, or submit_form. The upper camel case turns out to be the commonly used Pascal case.
- Consideration of the separate stateful aspects from rendering
The conditional rendering function in React is similar to JavaScript’s conditions. JavaScript creates items that describe the current state of the application or runs the precise conditional operator and let React adapt the UI to match them.function User (props) { return <h2>Check your profile</h2>; } function Stranger (props) { return <h2>Register yorself</h2>; }
Components in React have two forms-stateful or stateless. Stateful components play the role of storing information about the component’s state and providing the necessary context. The condition is the reverse in the case of stateless components. They have no memory and cannot give context to other parts of the UI.
The general rule is that they can just receive props (inputs) from the parent component and return u JSX elements. Such components become scalable and reusable, and similar to the pure function in JavaScript. One of ways to cater React best practices is possessing the stateful data-loading logic distinct from the versioning stateless logic. The best move, in this case, is that you can have one stateful component to load data and another stateless component that can help in displaying the data. The motto is the reduction of the complexity of the components.
- Default XSS protection with data binding
You may have encountered a situation where you needed to leverage the dangerouslySetInnerHTML property on a React element.const Markup = () => { const htmlstring = "<p>XSS safeguarding through explicit data binding</p>" return ( <div dangerouslySetInnerHTML={{ ___html: htmlstring }} /> }
Default XSS protection with data binding serves as a react best practices and Security standards. When learning more about the react architecture best practices, it’s worth noting that you do not forget to use the default data link with braces. In this case, React automatically evades values to protect you from XSS attacks. However, you can get this protection standard only when rendering.Also, make sure that you use JSX data-binding syntax {} to place data in your elements.
<div>{data}</div> is one of the prime examples. Also, note that you avoid dynamic attribute values without custom validation.
- Keeping a note of the Dangerous URLs
URLs that use the JavaScript protocol comprise of dynamic script material. Therefore, leverage validation to ensure that all of your URLs are either HTTP or https to help prevent JavaScript-based: URL script injection.URLs contain dynamic script content via javascript: protocol URLs. Make sure that you use validation to assure your links are http: or https: that can help avoid javascript: URL-based script injection. Achieve URL validation with the utilization of the native URL parsing function. The next role you need to play is to match the parsed protocol property to an allow list. Here is an example:function validateURL(url) { const parsed = new URL(url) return ['https:', 'http:'].includes(parsed.protocol) } <a href={validateURL(url) ? url : ''}>Click here!</a>
- Rendering HTML
With the application of the right concepts, React JS security is increasing. For example, you can add HTML directly into displayed DOM elements using the dangerously Set Inner HTML function.React JS security is improving with the utilization of the proper principles. Insert HTML directly into rendered DOM nodes with the use of the dangerouslySetInnerHTML. However, note that the content inserted this way must undergo sanitization beforehand.To make things better, the best move is to utilize a sanitization library like dompurify on any of the values prior to placing them into the dangerouslySetInnerHTML prop. Also, you can use dompurify when inserting HTML into the DOM:import purify from "dompurify"; <div dangerouslySetInnerHTML={{ __html:purify.sanitize(data) }} />
Read More:- Best React Chart Libraries
- Utilisation of the tools like Bit
One of React best practices helping to organize all your React components is tools like Bit. The motto behind using these tools is to maintain and reuse code. In addition to that, these tools also help code to become discoverable and promote team collaboration in building components.Maintaining and reusing code is the guiding principle behind employing these technologies. This is one of the tips for react developer. Additionally, these technologies encourage team cooperation while creating components and assist in making code discoverable. - Use of snippet libraries
Code snippets help keep up with the best and most recent syntax. The goal is also to keep your code relatively bug-free. That said, this practice serves as one of the React best practices that you should not miss out on.Many snippet libraries are already there that you can use, like, ES7 React, Redux, JS Snippets. Using snippet libraries is needed as the React architecture best practices also involve using code snippets. The use of code snippets makes it easy for you to keep up with the latest syntax. The best part is that the snippet libraries keep the code relatively bug-free.For instance, some extensions for Visual Studio Code significantly boost productivity. Snippet extensions are one category of these extensions.import React from 'react' const GoogleMap = () => { } export default GoogleMap
- Writing tests for all code
To write best react code, better create tests for the components for reducing mistakes. Testing guarantees that the parts behave as you would anticipate and reduces challenges for React Developers.When it comes to the implementation of any programming language, adequate testing is needed. The goal is to ensure that the new code added to your project integrates with the existing code. There are chances of breaking existing functionality. Writing tests for all codes can eradicate the issue. Create a _Test_ directory within the directory to conduct relevant tests.Divide tests in React into testing the functionality of components and tests on the application once it renders in the browser. Use cross-browser testing tools that can help with the objective of testing the latter category. For the former, use a JavaScript test runner, Jest, to help to emulate the HTML DOM using jsdom to test React components.A completely accurate test is only possible in a browser that has been long associated with the real device. Also, it can be said that Jest provides a good approximation of the real testing environment that can assist a developer a lot in the development phase of the project. Also, this principle serves as React practical advice for improving code quality. - Using Map Function for Dynamic Rendering of Arrays
The best way to get the expected results is to create an object with props that return a dynamic HTML block. You can do so without the need for writing repeated code. React provides a map() function that can serve as the best tool to display arrays in order. Using an array with a map() is one of the best ideas. Also, you can use one parameter from the array as a key.render () { let cartoons = [ "Pika", "Squi", "Bulb", "Char" ]; return ( <ul> {cartoons.map(name => <li key={name}>{name}</li>)} </ul> ); }
In addition to this, ES6 spread functions can send a whole list of parameters in an object by using Object.keys().
render () { let cartoons = { "Pika": { type: "Electric", level: 10 }, "Squi": { type: "Water", level: 10 }, "Bulb": { type: "Grass", level: 10 }, "Char": { type: "Fire", level: 10 } }; return ( <ul> {Object.keys(cartoons).map(name => <Cartoons key={name} {... cartoon[name]} />)} </ul> ); }
To dynamically render repeated HTML blocks, use map(). For instance, you can render a list of objects in li> tags using map().
const Items () => { = const arr = ["item1", "item2", "item3", "item4", "item5"]; return ( <> {arr.map((elem, index) => { <li key={elem+index}>{elem}</li>; })} </> ); };
Here is an example of how to present the list without a map for comparison (). This strategy uses a lot of repetition.
const List = () => { return ( <ul> <li>Item1</li> <li>Item2</li> <li>Item3</li> <li>Item4</li> <li>Item5</li> </ul> ); };
- Keeping state business logic separate from UI
Separate the state management logic from the UI logic that can help you in multiple ways. Components that do both are not impactful as they make them less reusable, more difficult to test, and difficult to refactor, especially when you’re looking for state management. Rather than writing the logic for placement of the state inside a component, the best move is to extract it into a hook of its own.and another child component that contains the user interface. Then, call the child inside while the adult hands over all the necessary props.function MyComponent() { const { value, handleClick, ... // Other imports } = useCustomHook() return ( <Element value={value} onClick={handleClick} /> }
- Embracing wishful thinking
A helpful technique to the program is the consideration of wishful thinking. There is a particular method that you need to follow for it. First of all, send an API request to fetch the users at the stage the component mounts. The next step is to show a placeholder until you’re getting a response back from the server.As soon as you get the response back, make sure that you show the list of the users. You wish here serves as the component called Fetch and is completely responsible for doing an API call. Also, it is associated with the list of query parameters. API call pending means that there is a need to show a placeholder.So, there is a need to pass a component called Placeholder that specifies how it appears. Once the API call finishes, automatically a component will be rendered. Keep building on top of the logic, adding more props to the Fetch component to specify pagination, sorting, filters.Send an API request to retrieve the users at the component mounting step first. Until you receive a response from the server, the following step is to display a placeholder. - The implementation of the Object-oriented React
React is all regarding functional programming. The introduction of hooks references the strong desire to shift away from components written as classes. The objective here is to enter the functional world. Functional programming is easy to reason about, and in addition to this objective, testing becomes easy. Try different techniques and notice how much they make sense.Create classes for the data that can assist you in the long run. For example, instead of json data, create a class like so: new Group(groupJsonDataFromApi). With that, also you can expect some added functionality.With that, you can also easily note the class for the Post entity and class for the Group entity. Also, the shared fields are prevalent, namely id and permissions. The best way to foster convenience is to also add getters for each class. The idea here is to test whether the authenticated user can delete a certain entity.React is based on JavaScript, an object-oriented language (albeit not quite comparable to languages like Java, C++, and many other classic Object-Oriented languages).class Text extends React.Component { render() { return <p>{this.props.children}</p>; } } React.render(<Text>Welcome to React World!</Text>, document.body);
- Learning the benefits of testing and doing it from the start
Writing automation tests comes with complexity in that, at a certain point, it’s impossible to test React projects manually without spending time and resources. When starting the project, it’s easy to justify skipping writing test code codebase in that circumstance becomes relatively small.However, when it comes to more than fifty components and multiple higher-order components, testing projects manually take a whole day. Writing test code serves the objective of making your code more modular. In addition to that, it also helps you find errors faster and safeguard against crashing. Automation testing helps grow projects when manual testing fails to verify the code.Testing should start early and work in tandem with development. Early detection of code errors through testing reduces future costs and prevents problems from arising during development or implementation. - Folder Layout
You must have to consider the folder layout to implementing the best practices for react js.React developer architecture’s reusable components are the main focus of the architecture, allowing the design pattern to be used by numerous internal projects. Therefore, the concept of an element react folder structure should be applied, according to which each component’s relevant files (such as JavaScript, CSS, or other assets) must be kept in its own folder.Components | --Sign In | --testing-- --Signin.test.js --Signin.jsx --Signin.scss --SigninAPI.js
This is an additional method for classifying the file kinds. In this, a single folder is used to store all files of the same type. For instance
APIs | --SigninAPI --ProfileAPI --UserAPI Components | --Signin.jsx --Signin.test.js --Profile.jsx --Profile.test.js --User.jsx
The fundamental example is the structure mentioned above. Depending on the needs, the folders might be nested even further.
- Higher-Order Components (HOC)
React’s advanced methodology enables for the reuse of component functionality within the render method. A component can be changed into a higher intelligence of the element by using an elevated version of the element.For instance, when the user logs in, we may want to display some components. You must include the exact identical code for each component to verify this. Here, the Higher-Order Component is used to ensure that the user is signed in and to keeping your code contained within a single app element. While this is wrapped around the other parts. - Use functional or class components
You can consider using functional components and it will be the best practices to learn React.Use functional components instead of class components if you only need to display the user interface without implementing any logic or changing the state of the system. Functional components are more effective in this situation. For illustration:// class component class Dog extends React.Component { render () { let { badOrGood, type, color } = this.props; return <div classname="{type}">My {color} Dog is { badOrGood } </div>; } } //function component let Dog = (badOrGood, type, color) => <div classname="{type}">My {color} Dog is { badOrGood }</div>;
Attempt to reduce the amount of functionality in React lifecycle actions like componentDidMount(), componentDidUpdate(), etc. These methods are not compatible with functional elements but may be used with Class components.
Utilizing functional components results in a loss of control over the rendering operation. It implies that the functional element constantly re-renders with a slight change to a component.
- Use Functional Components with Hooks
React v16.08’s new feature, “React Hooks,” makes it easy to create function components that interact with state. The complexity of handling states in Class components is decreased.Use functional components using React Hooks like useEffect(), useState(), etc. whenever possible. This will enable you to apply reasoning and facts frequently without making many changes to the hierarchical cycle. - Apply ES6 Spread Function
Using ES6 methods to send an object attribute would be a more straightforward and effective method. All of the object’s props will be automatically inserted if the phrase “…props” is used between the open and close tags.let propertiesList = { className: "selected-props ", id: "selected", content: "This is the selected props" }; let SmallDiv = props => <div {... props} />; let mainDiv = < SmallDiv props={propertiesList} />;
With the spread function, you can:
• There is no need for ternary operators.
• Only passing HTML tag attributes and content is not necessary.
When using functions repeatedly, avoid using the spread function in the following situations:
• Dynamic qualities exist.
• Array or object characteristics are required.
• When nested tags are necessary for rendering. - Manage Multiple Props with Parent/Child Component
Property management in components is challenging at any level, but with React’s state and ES6’s destructuring capability, props may be expressed more effectively, as illustrated below. For instance:Let’s build a programme that keeps track of saved locations and displays the GPS data of the user’s current position. As illustrated below, the location of the current user should be added to the favourite address and saved in the parent component app section.class App extends Component { constructor (props) { super(props); this.state = { currentUserLat: 0, currentUserLon: 0, isCloseToFavoriteAddress: false }; } }
We will now send at least two props from the app to gather information on how far current users are from their favourite address.
In the App’s render() method:<FavAddress ... // Information about the address addCurrentLat={this.state.currentUserLat} addCurrentLong={this.state.currentUserLon} />
For the FavAddress Component’s render():
render () { let { addHouseNumber, addStreetName, addStreetDirection, addCity, addState, addZip, addLat, addLon, addCurrentLat, addCurrentLon } = this.props; return ( ... ); }
It’s becoming cumbersome, as shown in the infographic up above. Keeping various sets of possibilities and separating them into their own individual entities is more practical.
- Take Out Extraneous Comments From the Code
Only add comments where they are necessary to avoid confusion when modifying the code later. Removing lines like Console.log, debugger, and unneeded commented code is also important. - Enhance HTTP Authentication with Security
In many applications, authentication is performed when a user logs in or creates an account. This procedure should be secure because client-side authentication and authorization might be vulnerable to numerous security flaws that may cause these protocols to be destroyed in the application. - Protect Against Faulty Authentication
The application may crash occasionally after you provide your authentication information, which could result in the misuse of your user credentials. In order to eliminate this type of vulnerability. - Safe from DDoS attacks
When the IPs are hidden and the entire application state management has flaws, security risks arise. This will limit the communication that the suspension of services may cause.
Key Takeaways
Building React applications at scale usually seems to be a complicated task that requires you to consider the best decision for the web developers. Best practice in React turns out to be the one that is associated with the users and your team.
The best tip is to experiment with tools and methods for scaling React projects. With that, it will be easier for you to go ahead with the react coding.
We at Technostacks, a leading React JS Development Company, have an experienced in working with the latest trends of front-end technologies. Contact us if you have any idea of implementing the react project.